Contour Plots#
import numpy as np
import matplotlib.pyplot as plt
Procedure#
The basic procedure to create a contour plot of a function \(z = f(x,y)\) over the intervals \(a \leq x \leq b\) and \(c \leq y \leq d\) is as follows:
Create a vector of \(x\) values from \(a\) to \(b\).
Create a vector of \(y\) values from \(c\) to \(d\).
Use
np.meshgrid
to create matrices \(X\) and \(Y\) of \(x\) and \(y\) values respectively.Compute matrix \(Z\) of values \(z_{j,i} = f(x_i,y_j)\).
Plot the result with
plt.contour
and specify the number of contour lines.Add a color bar and other figure properties.
For example, let’s create the contour plot of the function \(z = x^2 - xy + 2y^2 - 1\) over the intervals \(-2 \leq x \leq 2\) and \(-1 \leq y \leq 1\).
x = np.linspace(-2,2,51)
y = np.linspace(-1,1,51)
X,Y = np.meshgrid(x,y)
Z = X**2 - X*Y + 2*Y**2 - 1
plt.contour(X,Y,Z,levels=[-2,-1,0,1,2,3,4,5,6])
plt.grid(True), plt.colorbar()
plt.show()
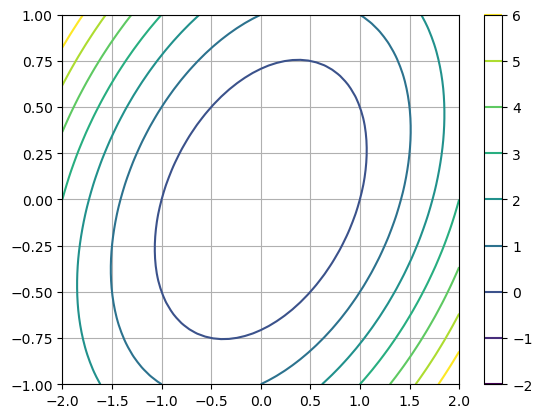
There are several other options:
Plot a filled contour plot with
plt.contourf
Choose a different colormap with
cmap
(see Matplotlib colormaps)Set the number of levels (as opposed to setting the \(z\) value of each level)
x = np.linspace(-2,2,51)
y = np.linspace(-1,1,51)
X,Y = np.meshgrid(x,y)
Z = X**2 - X*Y + 2*Y**2 - 1
plt.contourf(X,Y,Z,levels=20,cmap='jet')
plt.grid(True), plt.colorbar()
plt.show()
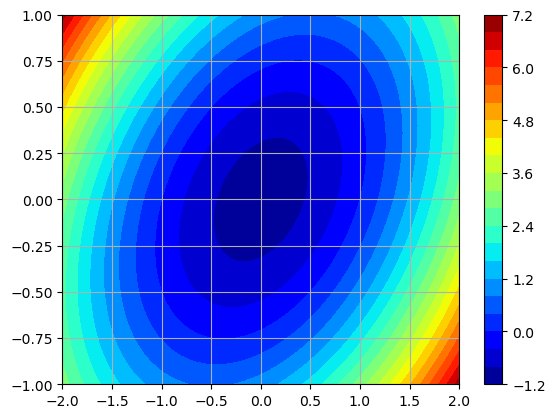
What is meshgrid
?#
The function np.meshgrid
takes vectors
and creates the matrices
Note that the rows of the matrix \(X\) are all equal to the vector \(\mathbf{x}\), and the columns of the matrix \(Y\) are all equal to the vector \(\mathbf{y}\). For example:
x = np.array([-2,-1,0,1,2])
y = np.array([0,0.5,1])
X,Y = np.meshgrid(x,y)
print(X)
[[-2 -1 0 1 2]
[-2 -1 0 1 2]
[-2 -1 0 1 2]]
print(Y)
[[0. 0. 0. 0. 0. ]
[0.5 0.5 0.5 0.5 0.5]
[1. 1. 1. 1. 1. ]]
What’s the point?!! Think about how we plot a function \(z = f(x,y)\):
Choose a vector of \(x\) values.
Choose a vector of \(y\) values.
Create the grid of points \((x_i,y_j)\).
Compute the values \(z_{ji} = f(x_i,y_j)\) at the grid points.
Connect adjacent points to create the surface!
The grid of points in the \(xy\)-plane is represented by the “matrix” of points:
Lets write out the matrix of \(z\) values:
Note the order of \(i\) and \(j\) in the notation: \(z_{j,i} = f(x_i,y_j)\). Therefore the matrix \(Z\) of \(z\) values is the result of applying the vectorized function \(f(x,y)\) to the matrices \(X\) and \(Y\).
In the end, all we need to do is copy/paste/modify the code above for new examples!
Examples#
Plot the function \(z = \cos(\pi x) + \sin(2 \pi y)\) over the intervals \(-2 \leq x \leq 2\) and \(-2 \leq y \leq 2\).
x = np.linspace(-2,2,101)
y = np.linspace(-2,2,101)
X,Y = np.meshgrid(x,y)
Z = np.cos(np.pi*X) + np.sin(2*np.pi*Y)
plt.contourf(X,Y,Z,cmap='magma')
plt.grid(True), plt.colorbar()
plt.show()
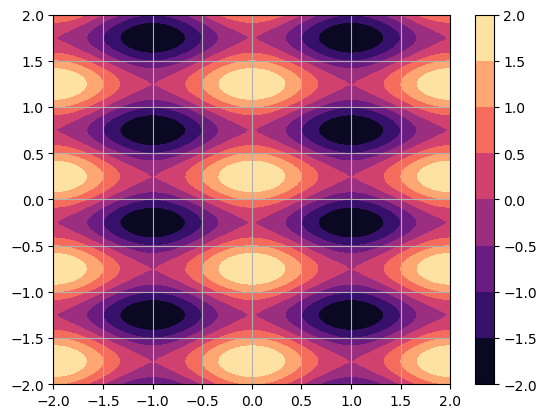
Plot the function \(z = (x^2 + 3y^2)e^{-x^2-y^2}\) over the intervals \(-2 \leq x \leq 2\) and \(-2 \leq y \leq 2\).
x = np.linspace(-2,2,101)
y = np.linspace(-2,2,101)
X,Y = np.meshgrid(x,y)
Z = (X**2 + 3*Y**2)*np.exp(-X**2-Y**2)
plt.contourf(X,Y,Z,levels=20,cmap='PuRd')
plt.grid(True), plt.colorbar()
plt.show()
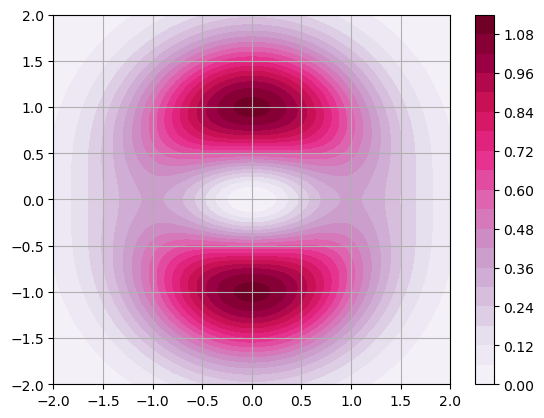