Finite Difference Method#
import numpy as np
import scipy.linalg as la
import matplotlib.pyplot as plt
Consider a second order linear differential equation with boundary conditions
The finite difference method is:
Discretize the domain: choose \(N\), let \(h = (t_f - t_0)/(N+1)\) and define \(t_k = t_0 + kh\).
Let \(y_k \approx y(t_k)\) denote the approximation of the solution at \(t_k\).
Substitute finite difference formulas into the equation to define an equation at each \(t_k\).
Rearrange the system of equations into a linear system \(A \mathbf{y} = \mathbf{b}\) and solve for $\( \mathbf{y} = \begin{bmatrix} y_1 & y_2 & \cdots & y_N \end{bmatrix}^T \)$
For example, consider an equation of the form
Choose \(N\), let \(h = (t_f - t_0)/(N + 1)\) and Llet \(r_k = r(t_k)\). The finite difference method yields the linear system \(A \mathbf{y} = \mathbf{b}\) where
Example 1#
Plot the approximation for the equation
and compare to the exact solution
t0 = 0; tf = 1; alpha = 0; beta = 0;
N = 4; h = (tf - t0)/(N + 1)
t = np.linspace(t0,tf,N+2)
r = -2*np.ones(t.size)
A = np.diag(np.repeat(-2,N)) + np.diag(np.repeat(1,N-1),1) + np.diag(np.repeat(1,N-1),-1)
b = h**2*r[1:N+1] - np.concatenate([alpha,np.zeros(N-2),beta],axis=None)
y = la.solve(A,b)
y = np.concatenate([alpha,y,beta],axis=None)
plt.plot(t,y,'b.-')
T = np.linspace(t0,tf,100)
Y = T*(1 - T)
plt.plot(T,Y,'r')
plt.grid(True)
plt.show()
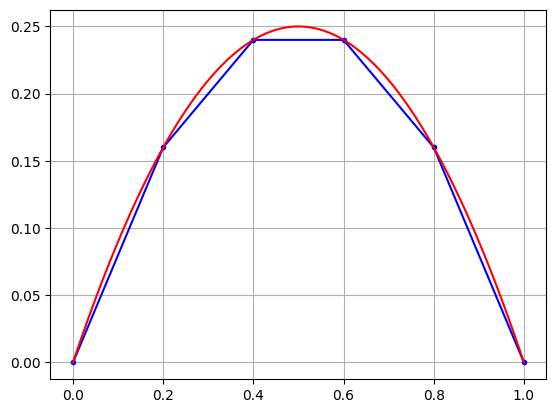
Example 2#
Plot the approximation for the equation
and compare to the exact solution
t0 = 0; tf = 2*np.pi; alpha = 0; beta = 1;
N = 5; h = (tf - t0)/(N + 1);
t = np.linspace(t0,tf,N+2)
r = np.cos(t)
A = np.diag(np.repeat(-2,N)) + np.diag(np.repeat(1,N-1),1) + np.diag(np.repeat(1,N-1),-1)
b = h**2*r[1:N+1] - np.concatenate([alpha,np.zeros(N-2),beta],axis=None)
y = la.solve(A,b)
y = np.concatenate([alpha,y,beta],axis=None)
plt.plot(t,y,'b.-')
T = np.linspace(t0,tf,100)
Y = 1-np.cos(T) + T/2/np.pi
plt.plot(T,Y,'r')
plt.grid(True)
plt.show()
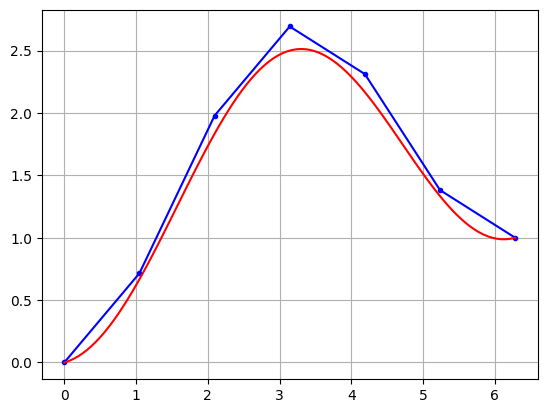
Example 3#
Consider a general second order linear ordinary differential equation with boundary conditions
Introduce the notation
The finite difference method yields the linear system \(A \mathbf{y} = \mathbf{b}\) where
Plot the approximation for the equation
alpha = 0; beta = 0; N = 19;
t0 = 0; tf = 3; h = (tf - t0)/(N + 1);
t = np.linspace(t0,tf,N+2)
p = t**2
q = np.ones(t.size)
r = np.cos(t)
a = 1 - h*p/2
b = h**2*q - 2
c = 1 + h*p/2
A = np.diag(b[1:N+1]) + np.diag(c[1:N],1) + np.diag(a[2:N+1],-1)
b = h**2*r[1:N+1] - np.concatenate([a[1]*alpha,np.zeros(N-2),c[N]*beta],axis=None)
y = la.solve(A,b)
y = np.concatenate([alpha,y,beta],axis=None)
plt.plot(t,y,'b.-')
plt.grid(True)
plt.show()
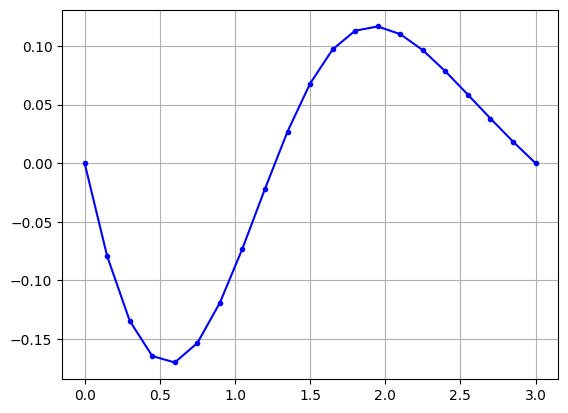