LU Decomposition#
Record the row operations of the Gaussian elimination algorithm in the LU decomposition and use the decomposition \(A = LU\) in backward/forward subsitution to efficiently solve a system of linear equations \(A \boldsymbol{x} = \boldsymbol{b}\).
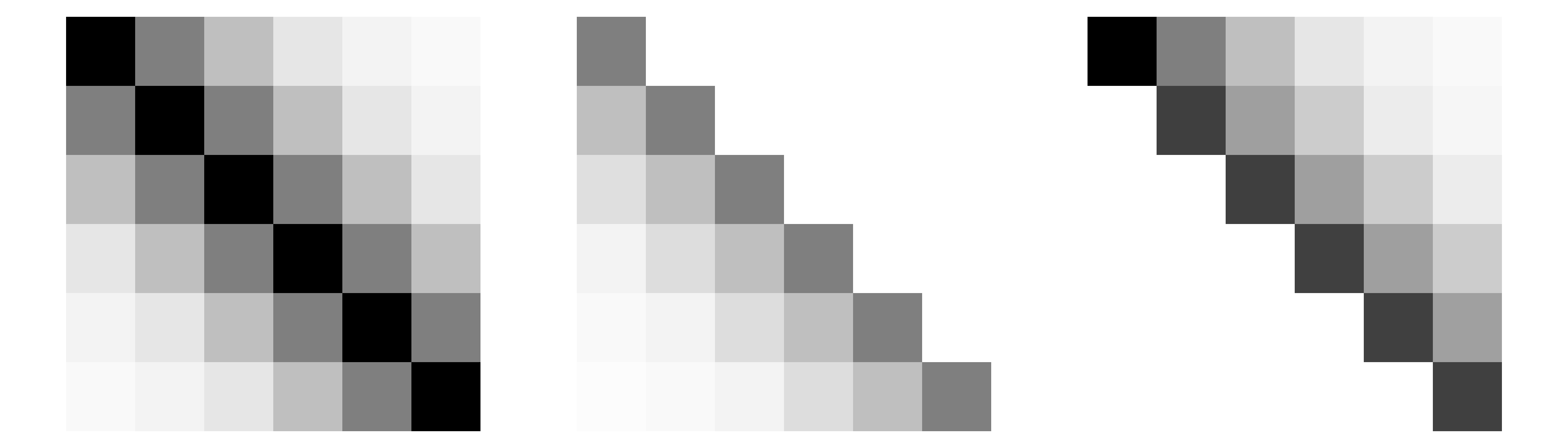
Gaussian Elimination#
A linear system of equations is a collection of equations of the form
where the coefficients \(a_{i,j}\) and \(b_i\) are known constants and \(x_i\) are unknown variables. In matrix notation, a linear system is \(A \boldsymbol{x} = \boldsymbol{b}\) where
There are three types of elementary row operations are:
Interchange two rows.
Multiply a row by a nonzero number.
Add a multiple of a row to another row.
A matrix is in row echelon form if:
All zero rows are at the bottom.
The first nonzero entry in a row (from the left) is located to the right of the first nonzero entry in every row above.
The following matrices are in row echelon form:
Symbol \(\times\) denotes a nonzero entry and \(*\) is any value.
The Gaussian elimination algorithm applies a sequence of elementary row operations to transform a matrix to row echelon form. See Wikipedia:Gaussian Elimination to review how the algorithm works.
Let \(A\) be a matrix and let \(U\) be a matrix in row echelon form obtained from \(A\) through a sequence of elementary row operations. The rank of \(A\) is the number of nonzero rows in \(U\). Denote the rank of a matrix \(A\) by \(\mathrm{rank}(A)\).
Let \(A\) be a \(m \times n\) matrix with \(\mathrm{rank}(A) = r\).
The system \(A \boldsymbol{x} = \boldsymbol{b}\) is inconsistent (ie. no solution) if \(\mathrm{rank}(A) < \mathrm{rank}([ \, A \ \boldsymbol{b} \, ])\). In other words, the row echelon form of the augmented matrix \([ \, A \ \boldsymbol{b} \, ]\) has a row of the form
which implies \(0 = 1\).
The system has a unique solution when \(\mathrm{rank}(A) = \mathrm{rank}([ \, A \ \boldsymbol{b} \, ])\) and \(\mathrm{rank}(A) = n\). In other words, the system is consistent and the rank of \(A\) is equal to the number of variables in the system.
The system has infinitely many solutions when \(\mathrm{rank}(A) = \mathrm{rank}([ \, A \ \boldsymbol{b} \, ])\) and \(\mathrm{rank}(A) < n\).
Find all solutions of \(A \boldsymbol{x} = \boldsymbol{b}\) where
Add -1 times row 1 to row 2, and add -2 times row 1 to row 3:
Add 3 times row 2 to row 3:
Since there is no leading one in column 3, assign the free variable \(t\) to \(x_3\). The second row implies \(x_2 = 1\) and the first row gives us
There are infinitely many solutions
Find all solutions of \(A \boldsymbol{x} = \boldsymbol{b}\) where
Add row 1 to row 2, add row 2 to row 3 and add -1 times row 1 to row 4:
Move row 2 to the bottom:
Add -2 times row 3 to row 4:
There is a unique solution
Find all solutions of \(A \boldsymbol{x} = \boldsymbol{b}\) where
Add -1 times row 1 to row 2:
Add row 2 to row 3:
The systems is inconsistent. In other words, there is no solution.
LU Decomposition#
A lower triangular matrix is a matrix with zeros above the diagonal. For example:
A unit lower triangular matrix is a square matrix with ones on the diagonal and zeros above the diagonal. For example:
An upper triangular matrix is a matrix with zeros below the diagonal. For example:
A unit upper triangular matrix is a square matrix with ones on the diagonal and zeros below the diagonal. For example:
Let \(E\) be the \(m \times m\) matrix with ones along the diagonal, \(c\) in the entry at row \(i\) and column \(j\) with \(i > j\) and all other entries are zeros
Then, for any \(m \times n\) matrix \(A\), matrix multiplication \(EA\) applies to \(A\) the elementary row operation: add \(c\) times row \(j\) to row \(i\).
Furthermore, the inverse of \(E\) is given by
where \(-c\) is the entry at row \(i\) and column \(j\).
Consider the matrix
The elementary matrix which adds -1 times row 1 to row 4 is
Perform matrix multiplication to verify
If \(A\) can be reduced by Gaussian elimination to row echelon form only with operations “add \(c\) times row \(j\) to row \(i\)” (in other words, without scaling rows and without interchanging rows), then \(A\) has an LU decomposition of the form
where \(L\) is a unit lower triangular matrix and \(U\) is an upper triangular matrix. In particular, after performing Gaussian elimination on \(A\), the matrix \(U\) is the corresponding row echelon form of \(A\) and \(L\) is given by
where each entry corresponds to the elementary row operation “add \(c_{i,j}\) times row \(j\) to row \(i\)” performed during Gaussian elimination.
Proof
Gaussian elimination (without scaling rows and without interchanging rows) yields a sequence of elementary row operations of the form “add \(c_{i,j}\) times row \(j\) to row \(i\)” for each pair \((i,j)\) with \(i > j\). Let \(E_{i,j}\) be the corresponding elementary matrix for each \((i,j)\). Then
where \(U\) is in row echelon form. Rearrange the equation to get
Each matrix \(E_{i,j}^{-1}\) is the unit lower triangular matrix with entry \(-c_{i,j}\) at index \((i,j)\). In particular, we have
Then \(E_{m,m-2}^{-1}E_{m,m-1}^{-1}\) results in add \(-c_{m,m-2}\) times row \(m-2\) to row \(m\) and so
Applying all the corresponding rows operations in order yields the result
Compute the LU decomposition of
Add -1 times row 1 to row 2 and add -2 times row 1 to row 3
Add row 2 to row 3
In terms of elementary matrices, we have just shown that
Rearrange the equation to get
Combine the matrices as in the proof of the LU decomposition to find
Therefore \(A = LU\) where
Note that we can construct the matrix \(L\) directly from the list of operations:
Add -1 times row 1 to row 2.
Add -2 times row 1 to row 3.
Add 1 times row 2 to row 3.
Compute the LU decomposition of
Add -2 times row 1 to row 2 and add -1 times row 1 to row 3:
Add row 2 to row 4:
Add row 3 to row 4:
Therefore \(A = LU\) where
Suppose \(A\) has an LU decomposition \(A = LU\).
\(\mathrm{rank}(A) = \mathrm{rank}(U)\)
\(\det(A) = \det(U) = u_{1,1} \cdots u_{m,m}\) where \(u_{1,1} , \dots , u_{m,m}\) are the diagonal entries of \(U\).
Not all matrices have an LU decomposition. For example,
does not have an LU decomposition (why not?). However, if we allow partial pivoting (ie. interchanging rows during Gaussian elimination), then Gaussian elimination with partial pivoting computes for any matrix \(A\) a decomposition \(A = PLU\) where \(P\) is a permutation matrix, \(L\) is unit lower triangular and \(U\) is upper triangular. This is called the LU decomposition with partial pivoting and has similar computational advantages as the LU decomposition. See Wikipedia:LU decomposition.
Forward and Backward Substitution#
Let \(A = LU\) be the LU decomposition of \(A\), let \(\ell_{i,j}\) denote the entries of \(L\) and let \(u_{i,j}\) denote the entries of \(U\). Consider the system \(A \boldsymbol{x} = \boldsymbol{b}\) and let \(\boldsymbol{y} = U \boldsymbol{x}\).
Forward substitution is the process of solving the lower triangular system \(L \boldsymbol{y} = \boldsymbol{b}\) from top to bottom:
Backward substitution is the process of solving the upper triangular system \(U \boldsymbol{x} = \boldsymbol{y}\) from bottom to top:
Solve the system \(A \boldsymbol{x} = \boldsymbol{b}\) where
Solve \(L \boldsymbol{y} = \boldsymbol{b}\)
and then solve \(U \boldsymbol{x} = \boldsymbol{y}\)
Therefore
The LU decomposition is especially useful when solving many different systems with the same coefficient matrix \(A\). For example, to compute the inverse \(A^{-1}\) of a square matrix of size \(n\) we need to solve \(n\) different systems \(A \boldsymbol{x}_k = \boldsymbol{e}_k\) for \(k=1,\dots,n\) where \(\boldsymbol{e}_k\) is the \(k\)th column of the identity matrix \(I\). The result is \(A^{-1} = [\boldsymbol{x}_1 \cdots \boldsymbol{x}_n]\). In other words, the columns of \(A^{-1}\) are given by \(\boldsymbol{x}_1, \dots, \boldsymbol{x}_n\).
Exercises#
Let \(A\) be a \(m\) by \(n\) matrix. Determine whether the statement is True or False.
If \(m > n\) and \(\mathrm{rank}(A) = n\), then here is a unique solution of \(A \boldsymbol{x} = \boldsymbol{b}\) for any \(\boldsymbol{b}\).
If \(m < n\) and \(\mathrm{rank}(A) = m\), then there are infinitely many solutions of \(A \boldsymbol{x} = \boldsymbol{b}\) for any \(\boldsymbol{b}\).
If \(m > n\) and \(\mathrm{rank}(A) = n\), then if the system \(A \boldsymbol{x} = \boldsymbol{b}\) has one solution then there is only one solution.
If \(m > n\) and \(\mathrm{rank}(A) < n\), then if the system \(A \boldsymbol{x} = \boldsymbol{b}\) has one solution then there are infinitely many solutions.
If \(A = LU\) is the LU decomposition of \(A\) then \(\det(L) \not= 0\).
Solution
False
True
True
True
True
Determine whether the statement is True or False. If \(A\) is of the form
and the \(LU\) decomposition \(A = LU\) exists, then \(L\) and \(U\) are of the form
Solution
True
Let \(I\) be the identity matrix of size \(n\) and let \(R\) be the \(n\) by \(n\) matrix with all zeros except for the nonzero scalar \(c\) at row \(i\) and column \(j\) where \(i \not= j\). Let \(E = I + R\) and let \(A\) be any \(n\) by \(n\) matrix.
Matrix multiplication \(EA\) is equivalent to which elementary row/column operation on \(A\)?
Matrix multiplication \(AE\) is equivalent to which elementary row/column operation on \(A\)?
Solution
Add \(c\) times row \(j\) to row \(i\).
Add \(c\) times column \(i\) to column \(j\).
Find a value \(c\) such that the system \(A \boldsymbol{x} = \boldsymbol{b}\) has infinitely many solutions where
Solution
\(c = 1\)
Compute the LU decomposition of the matrix
Solution
Consider the matrix
Find the \(LU\) decomposition of \(A\) and compute \(\mathrm{det}(A)\).
Solution
Find the solution of the system \(A \boldsymbol{x} = \boldsymbol{b}\) for
given the LU decomposition
Solution
Suppose we compute a decomposition \(A = L_0U_0\) such that \(U_0\) is unit upper triangular and \(L_0\) is lower triangular. Describe a method to derive a decomposition \(A = LU\) such that \(L\) is unit lower triangular and \(U\) is upper triangular.
Solution
Factor of the diagonal entries of \(L_0\) into a diagonal matrix \(D\) such that \(L_0 = LD\) where \(L\) is unit lower triangular then let \(U = DU_0\) to find \(A = LU\). For example: