Euler’s 3-Body Problem#
import numpy as np
import matplotlib.pyplot as plt
import scipy.integrate as spi
Problem Statement#
How does a planet move under the force of gravity due to two stars fixed in space?
See also
Check out Wikipedia: Euler’s 3-Body Problem for more information.
Variables and Parameters#
Description |
Symbol |
Dimensions |
Type |
---|---|---|---|
position of planet in \(x\)-direction |
\(x\) |
L |
dependent variable |
position of planet in \(y\)-direction |
\(y\) |
L |
dependent variable |
time |
\(t\) |
T |
independent variable |
mass of planet |
\(m_p\) |
M |
parameter |
mass of star 1 |
\(m_1\) |
M |
parameter |
mass of star 2 |
\(m_2\) |
M |
parameter |
gravitational constant |
\(G\) |
L3T-2M-1 |
parameter |
distance of each star from the origin |
\(d\) |
L |
parameter |
Assumptions and Constraints#
planet moves in \(xy\)-plane only
star 1 is located on the \(x\)-axis at \((-d,0)\)
star 2 is located on the \(x\)-axis at \((+d,0)\)
Model Construction#
The unit vector that points from the planet to star 1 is
and the unit vector that points from the planet to star 2 is
The sum of the gravitational forces of both stars acting on the planet is
Apply Newton’s second law of motion to get a second order, 2-dimenisional, nonlinear system of differential equations
with initial conditions
Apply nondimensionalization procedure. Let \(x = [x]x^*\), \(y = [y]y^*\) and \(t = [t]t^*\). It will simplify the equations greatly if we choose the same scaling factor \([c] = [x] = [y]\) for \(x\) and \(y\). Make the substitutions
Divide by highest order term and simplify
where \(d^* = d/[c]\). There are many choices and we choose \([c] = d\) and \([t] = \sqrt{\frac{d^3}{Gm_1}}\) and we arrive at
where \(\mu = \frac{m_2}{m_1}\).
Introduce new variables \(u_0 = x^*\), \(u_1 = \frac{dx}{dt^*}\), \(u_2 = y\), and \(u_3 = \frac{dy}{dt^*}\) to find
Plot some trajectories!
mu = 1.
def f(u,t):
dudt = np.array([0.,0.,0.,0.])
D1 = ((u[0] + 1)**2 + u[2]**2)**(3/2)
D2 = ((u[0] - 1)**2 + u[2]**2)**(3/2)
dudt[0] = u[1]
dudt[1] = -(u[0] + 1)/D1 - mu*(u[0] - 1)/D2
dudt[2] = u[3]
dudt[3] = -u[2]/D1 - mu*u[2]/D2
return dudt
u0 = [0.,1.5,1.,0.]
t = np.linspace(0,200,1000)
u = spi.odeint(f,u0,t)
plt.plot(u[:,0],u[:,2],-1,0,'r*',1,0,'r*'), plt.grid(True)
plt.show()
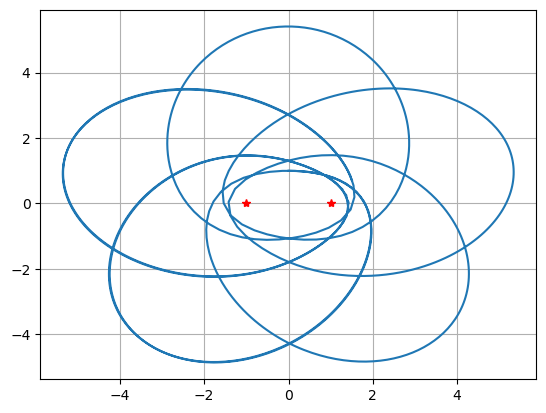
Analysis#
Under construction