Planetary Orbits#
import numpy as np
import matplotlib.pyplot as plt
import scipy.integrate as spi
Problem Statement#
How does a planet move around a star under the force of gravity?
Variables and Parameters#
Description |
Symbol |
Dimensions |
Type |
---|---|---|---|
position of planet in \(x\)-direction |
\(x\) |
L |
dependent variable |
position of planet in \(y\)-direction |
\(y\) |
L |
dependent variable |
time |
\(t\) |
T |
independent variable |
mass of the planet |
\(m_p\) |
M |
parameter |
mass of the star |
\(m_s\) |
M |
parameter |
gravitational constant |
\(G\) |
L3T-2M-1 |
parameter |
Assumptions and Constraints#
planet moves in the \(xy\)-plane only
the position of the star is fixed at the origin
no drag, friction or damping
planet starts on the \(x\)-axis at \(y(0) = 0\)
planet starts with velocity in \(y\)-direction only, \(x'(0) = 0\)
Model Construction#
The unit vector that points from the planet to the star is
The gravitational force vector acting on the planet is given by
Apply Newton’s second law of motion to get a second order, 2-dimenisional, nonlinear system of differential equations
with initial conditions
Apply nondimensionalization procedure. Let \(x = [x]x^*\), \(y = [y]y^*\) and \(t = [t]t^*\). The symmetry of the equations suggests that we should choose the same scaling factor \([c] = [x] = [y]\) for \(x\) and \(y\). Make the substitutions
Divide by highest order term and simplify
There are several choices we can make and so we choose
and we get
Introduce new variables \(u_0 = x^*\), \(u_1 = \frac{dx}{dt^*}\), \(u_2 = y\), and \(u_3 = \frac{dy}{dt^*}\) to find
Plot some trajectories!
def f(u,t):
dudt = np.array([0.,0.,0.,0.])
d3 = np.sqrt(u[0]**2 + u[2]**2)**3
dudt[0] = u[1]
dudt[1] = -u[0]/d3
dudt[2] = u[3]
dudt[3] = -u[2]/d3
return dudt
u0 = [1.,0.,0.,1.1]
t = np.linspace(0,10,200)
u = spi.odeint(f,u0,t)
plt.plot(u[:,0],u[:,2],0,0,'r*'), plt.grid(True), plt.axis('equal')
plt.show()
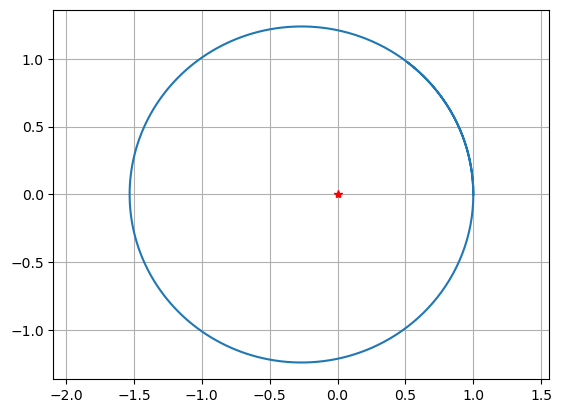
Analysis#
Under construction