Projectile Motion with Drag#
import numpy as np
import scipy.integrate as spi
import matplotlib.pyplot as plt
Problem Statement#
Construct a mathematical model of an object shot into the air and subject to the forces of drag and gravity.
Variables and Parameters#
Description |
Symbol |
Dimensions |
Type |
---|---|---|---|
position of projectile in \(x\)-direction |
\(x\) |
L |
dependent variable |
position of projectile in \(y\)-direction |
\(y\) |
L |
dependent variable |
time |
\(t\) |
T |
independent variable |
mass of the projectile |
\(m\) |
M |
parameter |
drag coefficient |
\(c\) |
MT-1 |
parameter |
constant of gravity |
\(g\) |
LT-2 |
parameter |
initial velocity |
\(v_0\) |
LT-1 |
parameter |
initial angle of velocity |
\(\theta\) |
1 |
parameter |
Assumptions and Constraints#
projectile moves in \(xy\)-plane only
initial position is at the origin \((0,0)\)
Construction#
The force of drag is proportional to velocity and acts in the direction oppposite the velocity
The force of gravity acts in the veritcal direction
Apply Newton’s second law to find the equations
Apply the nondimensionalization procedure. Let \(t = [t]t^*\), \(x = [x]x^*\) and \(y = [y]y^*\) and make the subsitution
Divide by second derivative term and simplify
Choose \([t] = \frac{m}{c}\) and \([x] = [y] = \frac{gm^2}{c^2}\) and write
where \(v_0^* = \frac{v_0c}{gm}\). These equations are independent of each other and can be solved analytically. However, we will approximate solutions using SciPy using the first order system with \(u_0 = x^*\), \(u_1 = \frac{dx^*}{dt^*}\), \(u_2 = y^*\), \(u_3 = \frac{dy^*}{dt^*}\):
Analysis#
def f(u,t):
dudt = np.zeros(4)
dudt[0] = u[1]
dudt[1] = -u[1]
dudt[2] = u[3]
dudt[3] = -1 - u[3]
return dudt
v0 = 10; theta = np.pi/6
u0 = [0,v0*np.cos(theta),0,v0*np.sin(theta)]
t = np.linspace(0,6,200)
u = spi.odeint(f,u0,t)
plt.plot(u[:,0],u[:,2]), plt.axis('equal'), plt.grid(True)
plt.show()
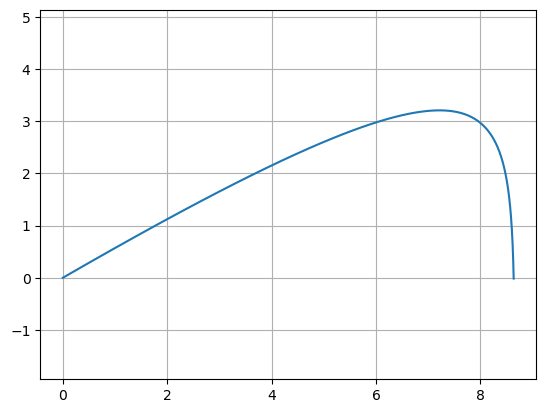